Want to send messages from your FiveM server to a Discord channel automatically? Webhooks are the way to go! They allow FiveM to send notifications, logs, and other messages directly into Discord.
Let’s go step by step to set it up. It’s easy, fun, and super useful!
What is a Discord Webhook?
A webhook is a special URL that lets external applications send data to a Discord channel. FiveM can use this to send messages whenever something happens in the game.
Step 1: Create a Discord Webhook
- Open Discord and go to your server.
- Find the channel where you want messages to appear.
- Click the gear icon next to the channel name.
- Go to the Integrations tab.
- Click Create Webhook.
- Give it a name and copy the webhook URL.
- Click Save Changes.
Awesome! Now you have a webhook URL. Time to use it with FiveM.
Step 2: Set Up Webhooks in Your FiveM Script
Now, let’s write a simple script that sends messages to Discord.
Using Lua
local webhookURL = "YOUR_WEBHOOK_URL_HERE"
function sendToDiscord(name, message, color)
local content = {
{
["title"] = name,
["description"] = message,
["color"] = color
}
}
PerformHttpRequest(webhookURL, function(err, text, headers) end, "POST", json.encode({embeds = content}), {["Content-Type"] = "application/json"})
end
sendToDiscord("Server Alert", "FiveM Server is Online!", 65280)
Save this as a Lua script inside your FiveM server files. Make sure to replace YOUR_WEBHOOK_URL_HERE
with the actual webhook URL you copied from Discord.
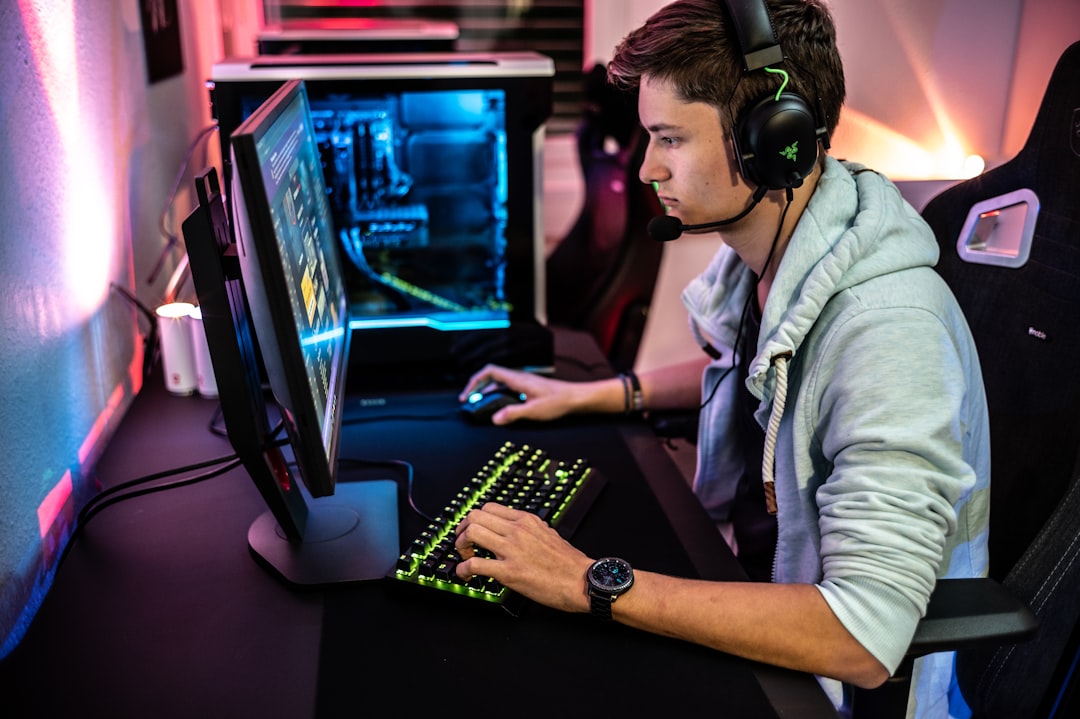
Step 3: Trigger Webhooks on Events
You can trigger webhook messages when players join, leave, or do actions.
AddEventHandler("playerConnecting", function(name, setKickReason, deferrals)
sendToDiscord("Player Joined", name .. " has joined the server!", 16711680)
end)
AddEventHandler("playerDropped", function(reason)
local name = GetPlayerName(source)
sendToDiscord("Player Left", name .. " left the server. Reason: " .. reason, 16776960)
end)
This will send alerts to Discord whenever a player joins or leaves the server.
Step 4: Testing Your Webhooks
Restart your FiveM server and watch the magic happen! Try joining and leaving the server. If everything is set up correctly, messages should appear in the Discord channel.
If it’s not working, check the following:
- Did you copy the webhook URL correctly?
- Are there any errors in the FiveM server console?
- Did you restart the server after adding the script?
Step 5: Customize Your Messages
Want stylish messages? Let’s add more details to the webhook message.
function sendToDiscord(name, message, color, footer)
local content = {
{
["title"] = name,
["description"] = message,
["color"] = color,
["footer"] = {
["text"] = footer
}
}
}
PerformHttpRequest(webhookURL, function(err, text, headers) end, "POST", json.encode({embeds = content}), {["Content-Type"] = "application/json"})
end
sendToDiscord("Server Alert", "A new event started!", 3447003, "Sent from FiveM Server")
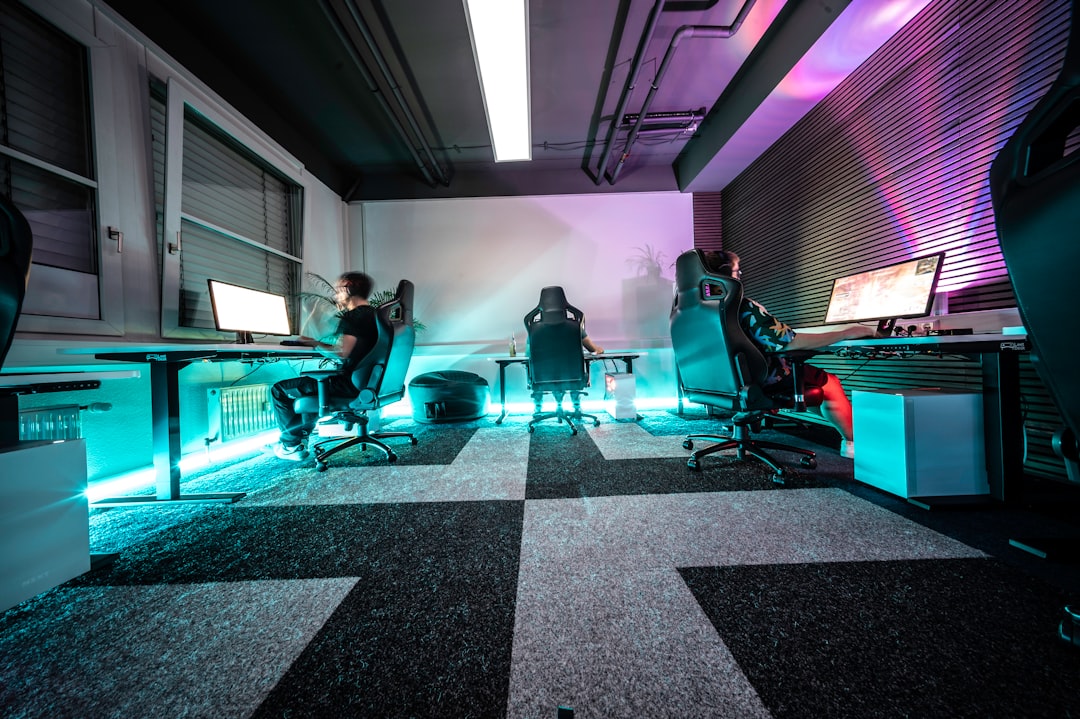
Extra Tips!
- You can add images and timestamps to messages.
- Use different webhooks for different types of messages (logs, announcements, etc.).
- Try integrating webhooks with other scripts, like player logs or in-game actions.
Conclusion
Discord webhooks are a great way to keep track of what’s happening on your FiveM server. They are fast, simple to set up, and can be customized to fit your needs.
Give it a try, and have fun automating your server notifications!